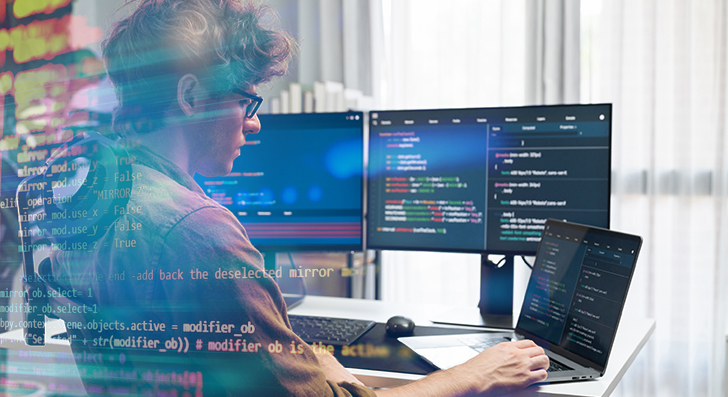
Scalability indicates your software can cope with expansion—a lot more customers, extra knowledge, and a lot more site visitors—with out breaking. As a developer, setting up with scalability in mind will save time and pressure later. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Quite a few programs are unsuccessful once they develop rapid simply because the first design and style can’t tackle the extra load. To be a developer, you should Imagine early about how your system will behave stressed.
Get started by planning your architecture to be versatile. Prevent monolithic codebases exactly where anything is tightly related. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial sections. Each module or support can scale By itself with out impacting The full procedure.
Also, consider your database from day one particular. Will it have to have to handle a million people or just a hundred? Choose the appropriate style—relational or NoSQL—based on how your info will increase. System for sharding, indexing, and backups early, Even when you don’t need to have them nonetheless.
Another essential level is to stop hardcoding assumptions. Don’t generate code that only works below existing problems. Consider what would occur Should your consumer base doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style designs that help scaling, like information queues or event-driven techniques. These support your application cope with additional requests without obtaining overloaded.
Once you build with scalability in mind, you're not just preparing for achievement—you are minimizing foreseeable future complications. A properly-planned process is simpler to keep up, adapt, and develop. It’s much better to arrange early than to rebuild afterwards.
Use the best Database
Deciding on the appropriate databases is really a vital Component of building scalable programs. Not all databases are constructed exactly the same, and using the Improper one can slow you down or even induce failures as your application grows.
Begin by comprehending your data. Can it be hugely structured, like rows inside a desk? If Of course, a relational databases like PostgreSQL or MySQL is a superb in shape. These are typically solid with interactions, transactions, and consistency. They also aid scaling tactics like go through replicas, indexing, and partitioning to manage a lot more traffic and knowledge.
In case your facts is more versatile—like user action logs, product catalogs, or paperwork—take into account a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with significant volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more easily.
Also, look at your study and produce designs. Are you currently executing lots of reads with less writes? Use caching and skim replicas. Have you been managing a major compose load? Take a look at databases that will deal with large write throughput, and even function-centered information storage techniques like Apache Kafka (for momentary details streams).
It’s also intelligent to Feel forward. You may not require Innovative scaling options now, but deciding on a database that supports them means you won’t want to change later on.
Use indexing to hurry up queries. Prevent needless joins. Normalize or denormalize your info dependant upon your entry styles. And generally observe databases general performance when you mature.
To put it briefly, the correct database is dependent upon your app’s construction, speed wants, And the way you count on it to expand. Get time to pick properly—it’ll conserve a great deal of issues later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, just about every smaller delay adds up. Improperly penned code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s essential to Create effective logic from the start.
Start out by composing thoroughly clean, easy code. Stay clear of repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Resolution if a simple one will work. Keep your capabilities limited, focused, and straightforward to test. Use profiling resources to uncover bottlenecks—spots in which your code takes far too extended to operate or makes use of an excessive amount of memory.
Future, examine your databases queries. These usually slow matters down a lot more than the code itself. Be sure Every question only asks for the info you really have to have. Stay away from Find *, which fetches every little thing, and in its place pick particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout significant tables.
In the event you detect the same info remaining requested time and again, use caching. Store the outcome quickly using resources like Redis or Memcached therefore you don’t have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and makes your app a lot more productive.
Make sure to take a look at with large datasets. Code and queries that function fantastic with one hundred data could crash every time they have to take care of 1 million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These steps help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more consumers and a lot more targeted traffic. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools aid keep your application speedy, secure, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than 1 server doing many of the do the job, the load balancer routes people to diverse servers depending on availability. What this means is no solitary server gets overloaded. If just one server goes down, the load balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this easy to build.
Caching is about storing info temporarily so it might be reused swiftly. When people request the same facts once again—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it from your cache.
There's two widespread sorts of caching:
1. Server-facet caching (like Redis or Memcached) shops details in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static files near to the person.
Caching lowers databases load, improves pace, and can make your application a lot more economical.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when facts does alter.
Briefly, load balancing and caching are simple but effective applications. With each other, they help your app manage additional users, remain rapid, and recover from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need instruments that let your application improve easily. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to purchase components or guess upcoming potential. When traffic increases, you are able to include much more sources with just a few clicks or immediately making use of automobile-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide services like managed databases, storage, load balancing, and security equipment. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and all the things it really should operate—code, libraries, options—into one device. This causes it to be straightforward to move your app read more concerning environments, from the laptop computer to the cloud, without the need of surprises. Docker is the preferred Device for this.
When your application makes use of numerous containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it immediately.
Containers also allow it to be easy to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container equipment means it is possible to scale quick, deploy quickly, and recover promptly when issues happen. If you need your application to expand without the need of limitations, start out utilizing these equipment early. They help you save time, minimize possibility, and assist you to keep centered on building, not repairing.
Watch Everything
In case you don’t observe your application, you won’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, place difficulties early, and make better choices as your app grows. It’s a essential Element of building scalable methods.
Start off by monitoring essential metrics like CPU use, memory, disk space, and response time. These let you know how your servers and providers are undertaking. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—watch your application much too. Regulate how much time it takes for users to load pages, how often errors happen, and where they happen. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. As an example, Should your response time goes over a limit or a company goes down, you'll want to get notified straight away. This can help you correct troubles quickly, frequently before users even see.
Checking is additionally helpful when you make variations. When you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back again prior to it results in authentic injury.
As your app grows, website traffic and info increase. Devoid of monitoring, you’ll miss indications of difficulty right until it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your process and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without the need of breaking under pressure. Start off compact, Feel major, and build clever.